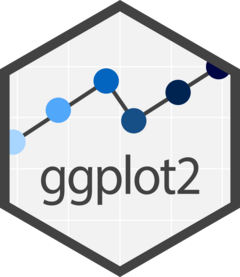
Calculate the element properties, by inheriting properties from its parents
Source:R/theme.R
calc_element.Rd
Calculate the element properties, by inheriting properties from its parents
Arguments
- element
The name of the theme element to calculate
- theme
A theme object (like
theme_grey()
)- verbose
If TRUE, print out which elements this one inherits from
- skip_blank
If TRUE, elements of type
element_blank
in the inheritance hierarchy will be ignored.
Examples
t <- theme_grey()
calc_element('text', t)
#> List of 11
#> $ family : chr ""
#> $ face : chr "plain"
#> $ colour : chr "black"
#> $ size : num 11
#> $ hjust : num 0.5
#> $ vjust : num 0.5
#> $ angle : num 0
#> $ lineheight : num 0.9
#> $ margin : 'margin' num [1:4] 0points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : logi FALSE
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
# Compare the "raw" element definition to the element with calculated inheritance
t$axis.text.x
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : NULL
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : num 1
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : 'margin' num [1:4] 2.2points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : NULL
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
calc_element('axis.text.x', t, verbose = TRUE)
#> axis.text.x -->
#> axis.text
#> axis.text -->
#> text
#> text -->
#> nothing (top level)
#> List of 11
#> $ family : chr ""
#> $ face : chr "plain"
#> $ colour : chr "grey30"
#> $ size : num 8.8
#> $ hjust : num 0.5
#> $ vjust : num 1
#> $ angle : num 0
#> $ lineheight : num 0.9
#> $ margin : 'margin' num [1:4] 2.2points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : logi FALSE
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
# This reports that axis.text.x inherits from axis.text,
# which inherits from text. You can view each of them with:
t$axis.text.x
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : NULL
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : num 1
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : 'margin' num [1:4] 2.2points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : NULL
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
t$axis.text
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : chr "grey30"
#> $ size : 'rel' num 0.8
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : NULL
#> $ debug : NULL
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
t$text
#> List of 11
#> $ family : chr ""
#> $ face : chr "plain"
#> $ colour : chr "black"
#> $ size : num 11
#> $ hjust : num 0.5
#> $ vjust : num 0.5
#> $ angle : num 0
#> $ lineheight : num 0.9
#> $ margin : 'margin' num [1:4] 0points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : logi FALSE
#> $ inherit.blank: logi TRUE
#> - attr(*, "class")= chr [1:2] "element_text" "element"